Understanding the `after()` Function in Next.js.
17.02.2025
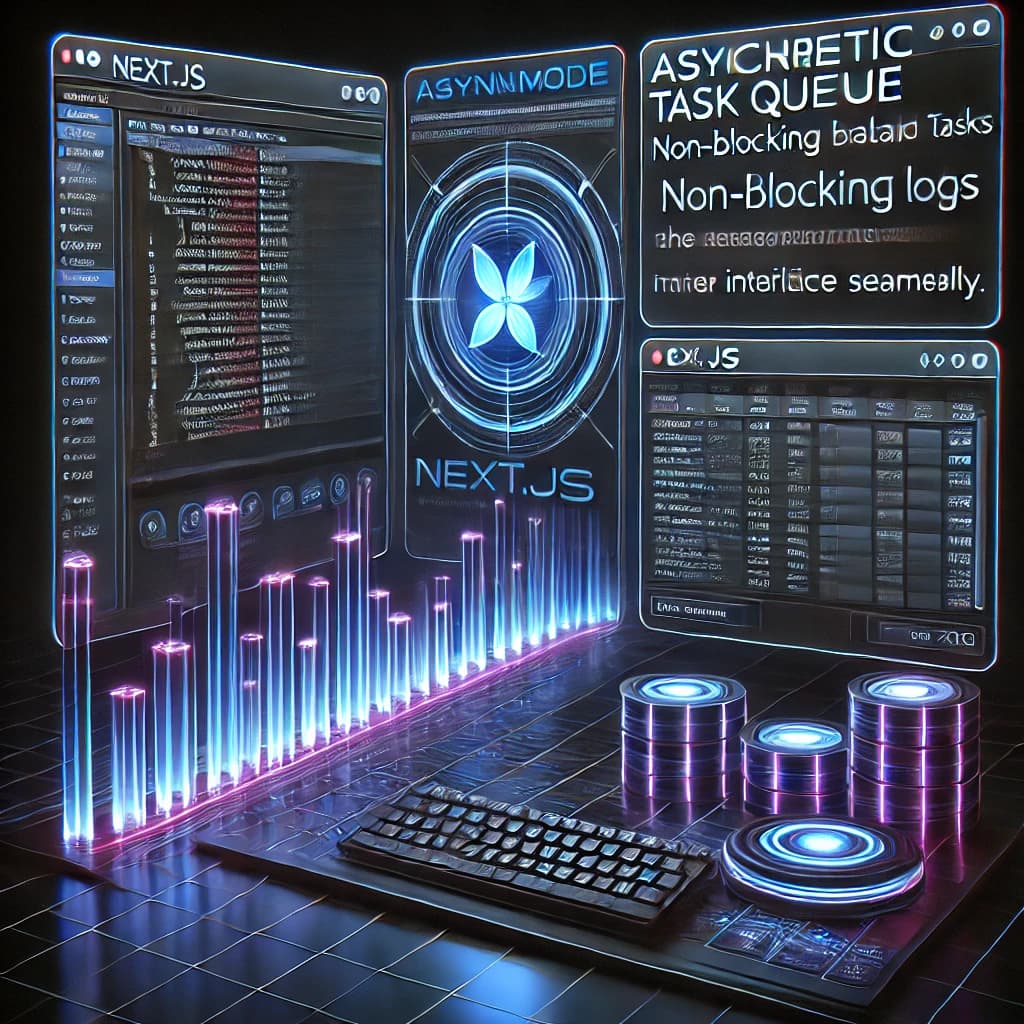
Introduction
In Next.js, the after()
function allows developers to schedule work that
should be executed after a response has been sent. This is particularly useful
for non-blocking side effects such as logging, analytics, and background tasks.
Without after()
, these operations could delay response times, forcing users to
wait unnecessarily. By leveraging after()
, developers can improve performance
while ensuring that necessary post-response operations are still executed.
Where Can after()
Be Used?
The after()
function can be utilized in the following scenarios:
- Server Components
- Server Actions
- Route Handlers
- Middleware
Example Usage
Consider a scenario where we want to log a page view but don’t want to block the
response. We can define a logging function and use after()
to execute it
asynchronously after the response is sent.
// lib/utils.ts
export async function log() {
await new Promise(resolve => setTimeout(resolve, 1000))
console.log('Page view logged')
}
Next, we integrate after()
within a server component:
import { after } from 'next/server'
import { log } from '@/lib/utils'
export default function Page() {
// Executes after response is sent
after(async () => await log())
return (
<div>
<h1>Welcome to My Page</h1>
</div>
)
}
Important Notes
after()
is not a Dynamic API. Callingafter()
does not make a route dynamic. If used within a static page, the callback will execute only at build time or during revalidation.- Executes Even on Errors – The
after()
callback runs even if the response fails, including cases where an error is thrown or whennoFound()
orredirect()
is called. - Deduplication with React Cache – You can use React's cache to prevent
redundant function calls within
after()
. - Nested Calls –
after()
can be nested within otherafter()
calls. - APIs Access Restrictions – In Server Actions and Route Handlers,
you can access APIs like cookies and headers within
after()
. However, in Server Components, this is not possible.
Alternative: waitUntil()
Vercel provides an alternative function, waitUntil()
, which schedules tasks
slightly differently:
Feature | after() | waitUntil() |
---|---|---|
Execution Time | After the response is sent | During the request lifecycle |
Accepts | A callback function | A promise |
Use Case Example: Authentication Middleware
A practical scenario for using after()
is tracking user activity without
blocking page rendering. For instance, when using Clerk for authentication,
we may want to log the user's last visit without delaying page load.
import { after } from 'next/server'
import { updateLastLogin } from '@/lib/auth'
export function middleware(req) {
after(async () => await updateLastLogin(req))
return NextResponse.next()
}
In this case, updateLastLogin()
runs after the middleware completes, ensuring
that the page loads quickly while still recording user activity.
Conclusion
The after()
function is a powerful tool in Next.js for executing background
tasks without affecting response times. It improves performance by ensuring that
non-essential operations occur asynchronously. By understanding its behavior and
alternatives like waitUntil()
, developers can make informed decisions on when
and how to use it effectively.