Mastering SEO in Next.js
17.02.2025
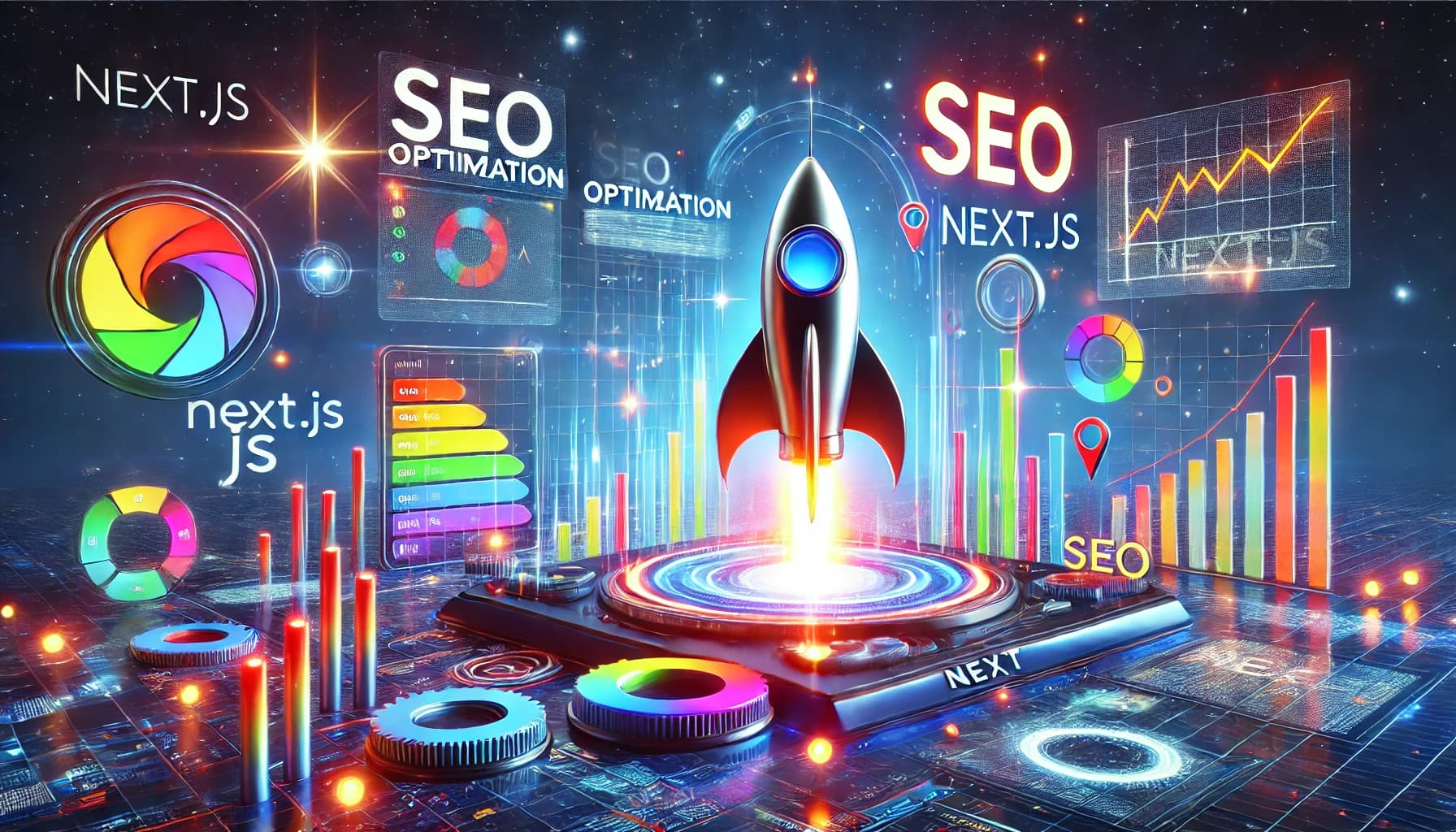
Introduction
Search Engine Optimization (SEO) is a crucial aspect of web development, and Next.js offers powerful tools to help you optimize your site for search engines. This guide will walk you through essential SEO techniques in Next.js, covering metadata, sitemap generation, robots.txt, rendering strategies, and best practices for indexing and analytics.
1. Metadata: Static and Dynamic
Metadata plays a critical role in how search engines and social media platforms interpret and display your website. In Next.js, you can define metadata both statically and dynamically.
Static Metadata Configuration
// layout.tsx
import type { Metadata } from 'next'
export const metadata: Metadata = {
title: {
template: '%s | Your Site Name',
default: 'Your Site Name'
},
description: 'A brief description of your website'
}
For individual pages, you can override the metadata:
// about/page.tsx
import type { Metadata } from 'next'
export const metadata: Metadata = {
title: 'About Us',
description: 'Learn more about our company and team'
}
Dynamic Metadata Using generateMetadata
For pages requiring dynamic metadata (e.g., blog posts), use the
generateMetadata
function:
// src/app/posts/[postId]/page.tsx
import { Metadata } from 'next'
import { notFound } from 'next/navigation'
interface BlogPostPageProps {
params: { postId: string }
}
export async function generateMetadata({
params: { postId }
}: BlogPostPageProps): Promise<Metadata> {
const response = await fetch(`https://dummyjson.com/posts/${postId}`)
const post: BlogPost = await response.json()
return {
title: post.title,
description: post.body
// openGraph: {
// images: [
// {
// url: post.imageUrl
// }
// ]
// }
}
}
export default async function BlogPostPage({
params: { postId }
}: BlogPostPageProps) {
const response = await fetch(`https://dummyjson.com/posts/${postId}`)
const { title, body }: BlogPost = await response.json()
if (response.status === 404) {
notFound()
}
return (
<article>
<h1>{title}</h1>
<p>{body}</p>
</article>
)
}
2. Open Graph & Favicon
Open Graph (OG) tags improve how your website appears when shared on social media.
- Recommended size: 1200x630px
- Supported formats: .jpg, .jpeg, .png, .gif
Save your Open Graph image as opengraph-image.png
in src/app/
.
This results in:
<meta property="og:image" content="<generated>" />
<meta property="og:image:width" content="1200" />
<meta property="og:image:height" content="630" />
Use tools like OpenGraph.xyz or Social Share Preview to verify OG metadata.
Favicon
Generate a favicon using a free online Favicon Generator
and place favicon.ico
in src/app/
.
3. Rendering & Caching Strategies
Static vs. Dynamic Rendering:
- Static Generation (SSG): Pre-render pages at build time for improved speed and SEO.
- Server-Side Rendering (SSR): Generate content dynamically for frequently updated pages.
Use caching to optimize data fetching and prevent redundant requests:
import { cache } from 'react'
const getPost = cache(async (postId: string) => {
const post = await fetch(`https://dummyjson.com/posts/${postId}`).then(res =>
res.json()
)
return post
})
For static content, pre-generate pages at build time:
export async function generateStaticParams() {
const posts = await fetch('https://dummyjson.com/posts').then(res =>
res.json()
)
return posts.map(({ id }: { id: string }) => ({ postId: id }))
}
Note:
if you use the native fetch function it will not deduplicate cache request. If you are using database, axios and such see the example below to prevent request duplication using React cache.
import type { Metadata } from 'next'
import { cache } from 'react'
interface BlogPostPageProps {
params: { postId: string }
}
// Manaully dedeuplicte a request if not using fetch
const getPost = cache(async (postId: string) => {
const post = await prisma.post.findUnique(postId)
return post
})
// Converting dynamic (server side redering) to static (prerendered static content). passing the Id's of the posts to render these pages at compile time. It compiles at compile time; fast loading and better seo.
export async function generateStaticParams() {
const posts = await getPosts()
const slugs = posts.map(({ id }) => id)
return slugs
}
export async function generateMetaData({
params: { postId }
}: BlogPostPageProps): Promise<Metadata> {
// const response = await fetch(`https://dummy/posts/${postId}`);
const response = await getPost(postId)
const post: BlogPost = await response.json()
return {
title: post.title,
description: post.body
}
}
export default async function BlogPostPage({
params: { postId }
}: BlogPostPageProps) {
// const response = await fetch(`https://dummy/posts/${postId}`);
const response = await getPost(postId)
const { title, body }: BlogPost = await response.json()
}
4. Sitemap and Robots.txt
A sitemap helps search engines index your site efficiently.
sitemap.(xml|js|ts)
is a special file that matches
the Sitemaps XML format to help search
engine crawlers index your site more efficiently.
Static Sitemap Example
**<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
<url>
<loc>https://yourwebsite.com</loc>
<lastmod>2024-01-01</lastmod>
<changefreq>yearly</changefreq>
<priority>1</priority>
</url>
</urlset>**
Dynamic Sitemap in Next.js
// app/sitemap.ts
import { BlogPosts } from '@/models/BlogPost'
import { MetadataRoute } from 'next'
export default async function sitemap(): Promise<MetadataRoute.Sitemap> {
const response = await fetch('https://dummyjson.com/posts')
const { posts }: BlogPosts = await response.json()
const postEntries: MetadataRoute.Sitemap = posts.map(({ id }) => ({
url: `${process.env.NEXT_PUBLIC_BASE_URL}/posts/${id}`
// lastModified: new Date(post.updatedAt),
// changeFrequency:,
// priority:
}))
return [
{
url: `${process.env.NEXT_PUBLIC_BASE_URL}/about`,
lastModified: new Date()
},
...postEntries
]
}
robots.txt
Robots.txt controls which pages search engines can crawl.
Static Robots.txt
// app/robots.txt
User-Agent: *
Allow: /
Disallow: /private/
Sitemap: https://yourwebsite.com/sitemap.xml
Dynamic Robots.ts
import type { MetadataRoute } from 'next'
export default function robots(): MetadataRoute.Robots {
return {
rules: [
{
userAgent: '*',
allow: '/',
disallow: '/private/'
}
],
sitemap: `${process.env.NEXT_PUBLIC_BASE_URL}/sitemap.xml`
}
}
5. Google Search Console & Analytics
Google Search Console
Google Search Console is a free tool that helps monitor your site's visibility on Google Search.
- Go to Google Search Console
- Add your website as a Property
- Verify your domain via DNS TXT record or URL prefix
- Submit your sitemap (e.g.,
https://yourwebsite.com/sitemap.xml
) - Use
site:yourwebsite.com
in Google to check indexed pages
Vercel Analytics
Vercel offers built-in analytics to track traffic and performance.
- Navigate to your Vercel project settings
- Enable Vercel Analytics
- Monitor real-time insights and performance data
Final Thoughts and more about Next.js and SEO optimization
Next.js is a powerful framework for SEO optimization. By implementing structured metadata, optimizing rendering strategies, and leveraging Google Search Console and analytics, you can significantly improve your site's visibility and performance.
By following these best practices, your Next.js website will not only be highly optimized for search engines but also deliver a superior user experience.
Next.js has emerged as a powerful framework for building search engine optimized websites, offering several key advantages for SEO implementation. At its core, Next.js utilizes server-side rendering (SSR), ensuring that web crawlers can efficiently discover and index your content.
One of the framework's standout features is its support for dynamic routes, which enables developers to target multiple keyword variations efficiently without manual page setup. This is complemented by Next.js's sophisticated caching system, which not only enhances page loading speeds but also supports incremental static regeneration, allowing for controlled content updates while maintaining optimal performance.
Implementation Strategy
The implementation process can be broken down into three key steps:
- Page Template Optimization: Create optimized templates that leverage existing data to generate statically cached, SEO-friendly pages.
- Automated Sitemap Generation: Implement a system that automatically generates and updates your sitemap to include all pages, eliminating the need for manual updates.
- Search Engine Submission: Complete the process by submitting your sitemap to Google, ensuring all your pages are properly indexed.
This streamlined approach ensures your Next.js application is not only search engine friendly but also maintains high performance standards while scaling.